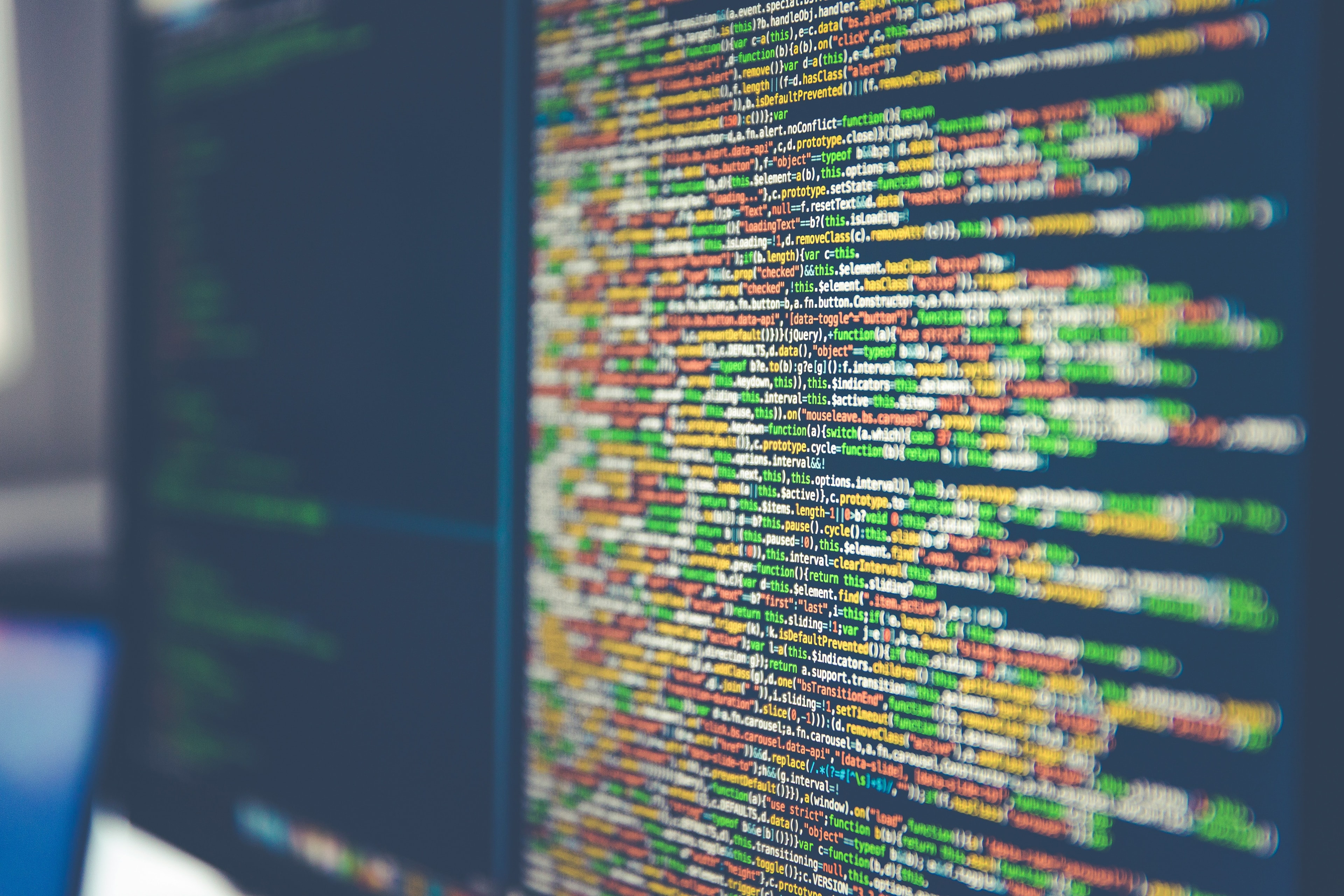
Building Scalable Microservices with NestJS and AWS
In this comprehensive guide, we'll explore how to build scalable microservices using NestJS and AWS serverless services.
Why NestJS?
NestJS provides a robust framework for building server-side applications. Its modular architecture makes it perfect for microservices.
Advantages of NestJS
- Modularity: Allows you to divide your application into reusable modules.
- TypeScript: Offers better type safety and code readability.
- Microservices Support: Easy integration with messaging systems like RabbitMQ and Kafka.
Why Use AWS for Microservices?
AWS (Amazon Web Services) provides a robust and scalable infrastructure for deploying applications. Here are some AWS services that are particularly useful for microservices:
- AWS Lambda: Runs code without managing servers, ideal for specific functions.
- Amazon API Gateway: Manages RESTful and WebSocket APIs, making it easy to create and manage endpoints.
- Amazon DynamoDB: Fully managed NoSQL database that offers low latency and automatic scaling.
Microservices Architecture
A typical microservices architecture consists of several independent services that communicate with each other via APIs. Here’s an example architecture:
- Authentication Service: Manages user authentication.
- User Management Service: Handles user information.
- Product Management Service: Manages product information.
Each service can be deployed independently, allowing for easier scaling and maintenance.
Setting Up a Microservice with NestJS
Step 1: Install NestJS
To get started, you need to install the NestJS CLI:
npm i -g @nestjs/cli
Create a new project:
nest new microservice-example
Step 2: Create a Microservice Module
Create a module for your microservice, for example, a user management service:
nest generate module users
nest generate service users
Step 3: Implement the Service
In the users.service.ts
file, you can add methods to manage users:
import { Injectable } from "@nestjs/common";
@Injectable()
export class UsersService {
private users = [];
create(user) {
this.users.push(user);
return user;
}
findAll() {
return this.users;
}
}
Step 4: Create a Controller
Create a controller to handle HTTP requests:
nest generate controller users
In the users.controller.ts
file, you can define the routes:
import { Controller, Get, Post, Body } from "@nestjs/common";
import { UsersService } from "./users.service";
@Controller("users")
export class UsersController {
constructor(private readonly usersService: UsersService) {}
@Post()
create(@Body() user) {
return this.usersService.create(user);
}
@Get()
findAll() {
return this.usersService.findAll();
}
}
Step 5: Deploying on AWS
To deploy your microservice on AWS, you can use AWS Lambda and API Gateway. Here are the general steps:
- Create a Lambda Function: Go to the AWS Lambda console and create a new function.
- Configure API Gateway: Create a new REST API in API Gateway and configure the endpoints for your Lambda function.
- Deploy: Once everything is set up, deploy your API.
Best Practices for Microservices
- Error Handling: Implement robust error handling for each service.
- Monitoring and Logging: Use tools like AWS CloudWatch to monitor your services.
- Testing: Write unit and integration tests to ensure code quality.
Conclusion
Building scalable microservices with NestJS and AWS is a powerful approach to developing modern applications. By leveraging the features of NestJS and the infrastructure of AWS, you can create robust and scalable services that meet your business needs.