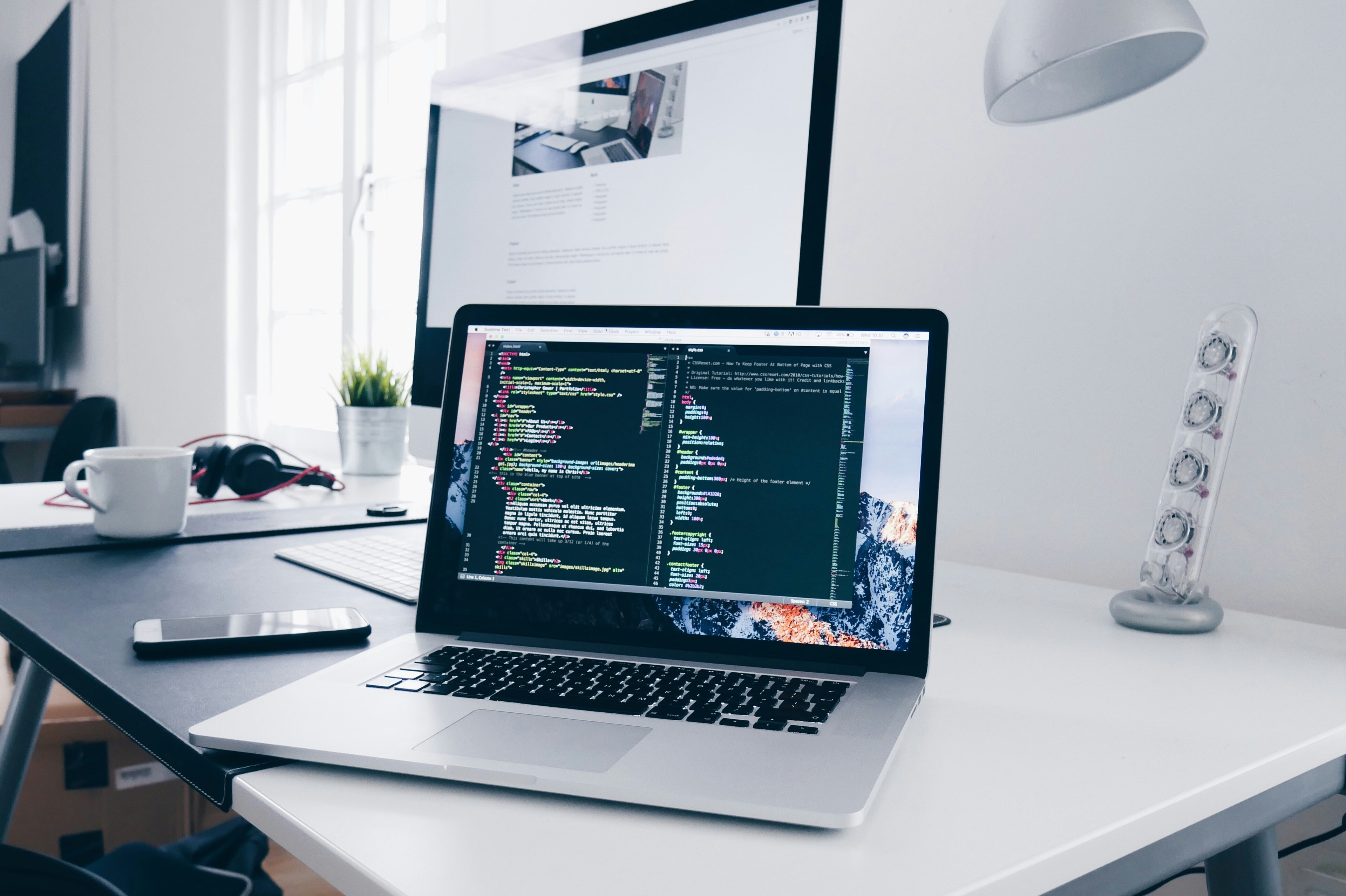
Mastering Form Management in Next.js: A Complete Guide
Table of Contents
- Introduction
- Modern Form Architecture
- Form Validation & Type Safety
- Advanced Form Patterns
- Performance Optimization
- Accessibility & UX
- Server Integration
- Testing Strategy
- Real-World Example: Multi-step Booking Form
- Best Practices & Common Pitfalls
Introduction
Forms are a crucial part of web applications, yet building them correctly with proper validation, error handling, accessibility, and good user experience can be challenging. This guide will show you how to build enterprise-grade forms in Next.js using modern tools and best practices.
Modern Form Architecture
Let's start by setting up a robust form architecture using React Hook Form, Zod, and custom hooks.
Base Form Setup
This implementation presents a robust form management system built with React Hook Form and Zod. Key features include:
- Type-safe form validation
- Efficient form state management
- Reusable form components
- Performance optimization strategies
Benefits:
- Reduced boilerplate code
- Improved type safety
- Better developer experience
- Enhanced performance
// lib/hooks/useForm.ts
import { zodResolver } from "@hookform/resolvers/zod";
import { useForm as useHookForm } from "react-hook-form";
import type { UseFormProps } from "react-hook-form";
import type { TypeOf, ZodSchema } from "zod";
interface UseFormOptions<T extends ZodSchema>
extends Omit<UseFormProps<TypeOf<T>>, "resolver"> {
schema: T;
}
export function useForm<T extends ZodSchema>({
schema,
defaultValues,
...formConfig
}: UseFormOptions<T>) {
return useHookForm({
...formConfig,
resolver: zodResolver(schema),
defaultValues,
});
}
// lib/validations/form.ts
import { z } from "zod";
export const formFieldSchema = z.object({
label: z.string(),
name: z.string(),
type: z.enum(["text", "email", "password", "number", "tel", "date"]),
required: z.boolean().default(false),
placeholder: z.string().optional(),
helperText: z.string().optional(),
validation: z.record(z.any()).optional(),
});
export type FormField = z.infer<typeof formFieldSchema>;
// components/ui/form.tsx
import * as React from "react";
import { useFormContext } from "react-hook-form";
import { cn } from "@/lib/utils";
import { Label } from "./label";
import { Input } from "./input";
interface FormFieldProps extends React.InputHTMLAttributes<HTMLInputElement> {
name: string;
label?: string;
helperText?: string;
}
export function FormField({
name,
label,
helperText,
className,
...props
}: FormFieldProps) {
const {
register,
formState: { errors },
} = useFormContext();
const error = errors[name];
return (
<div className="space-y-2">
{label && (
<Label htmlFor={name} className={cn(error && "text-destructive")}>
{label}
</Label>
)}
<Input
id={name}
className={cn(error && "border-destructive", className)}
{...register(name)}
{...props}
/>
{(helperText || error) && (
<p
className={cn(
"text-sm",
error ? "text-destructive" : "text-muted-foreground"
)}
>
{error ? error.message?.toString() : helperText}
</p>
)}
</div>
);
}
Form Validation & Type Safety
Advanced Validation Patterns
Form validation is critical for data integrity. This section covers:
- Complex validation rules with Zod
- Cross-field validation
- Async validation
- Custom validation messages
Key concepts:
- Schema-based validation
- Real-time validation
- Error handling
- Validation performance optimization
// lib/validations/booking.ts
import { z } from "zod";
// Custom validation functions
const isValidPhoneNumber = (value: string) => {
const phoneRegex = /^\+?[1-9]\d{1,14}$/;
return phoneRegex.test(value);
};
const isWorkingHours = (date: Date) => {
const hours = date.getHours();
return hours >= 9 && hours <= 17;
};
// Booking form schema
export const bookingFormSchema = z.object({
personalInfo: z.object({
firstName: z.string().min(2, "First name must be at least 2 characters"),
lastName: z.string().min(2, "Last name must be at least 2 characters"),
email: z.string().email("Invalid email address"),
phone: z.string().refine(isValidPhoneNumber, "Invalid phone number"),
}),
appointment: z.object({
date: z
.date()
.min(new Date(), "Date cannot be in the past")
.refine(isWorkingHours, "Please select a time between 9 AM and 5 PM"),
service: z.string().min(1, "Please select a service"),
notes: z.string().max(500, "Notes cannot exceed 500 characters").optional(),
}),
preferences: z.object({
reminders: z.boolean(),
reminderType: z.enum(["email", "sms"]).optional(),
marketingConsent: z.boolean(),
}),
});
export type BookingFormData = z.infer<typeof bookingFormSchema>;
Advanced Form Patterns
Multi-step Form Implementation
Multi-step forms require careful state management and user experience considerations:
- State persistence between steps
- Validation per step
- Navigation logic
- Progress tracking
- Data preservation
Implementation benefits:
- Improved user experience
- Better error handling
- Efficient state management
- Smooth transitions
// components/multi-step-form/use-multi-step-form.ts
import { useState } from "react";
import { useForm, UseFormReturn } from "react-hook-form";
import { z } from "zod";
interface UseMultiStepFormOptions<T extends z.ZodObject<any>> {
schema: T;
defaultValues?: z.infer<T>;
onSubmit: (data: z.infer<T>) => Promise<void>;
}
export function useMultiStepForm<T extends z.ZodObject<any>>({
schema,
defaultValues,
onSubmit,
}: UseMultiStepFormOptions<T>) {
const [currentStep, setCurrentStep] = useState(0);
const form = useForm<z.infer<T>>({
defaultValues,
resolver: zodResolver(schema),
mode: "onChange",
});
const next = async () => {
const isValid = await form.trigger();
if (isValid) setCurrentStep((prev) => prev + 1);
};
const back = () => {
setCurrentStep((prev) => prev - 1);
};
const goTo = (step: number) => {
setCurrentStep(step);
};
return {
currentStep,
form,
next,
back,
goTo,
isFirstStep: currentStep === 0,
isLastStep: currentStep === steps.length - 1,
};
}
// components/multi-step-form/form-step.tsx
interface FormStepProps {
title: string;
description: string;
children: React.ReactNode;
isActive: boolean;
}
export function FormStep({
title,
description,
children,
isActive,
}: FormStepProps) {
if (!isActive) return null;
return (
<div className="space-y-4">
<div className="space-y-2">
<h2 className="text-2xl font-bold tracking-tight">{title}</h2>
<p className="text-muted-foreground">{description}</p>
</div>
{children}
</div>
);
}
Performance Optimization
Form Performance Patterns
Performance is crucial for complex forms. This section demonstrates:
- Debounced validation
- Optimized re-renders
- Field-level updates
- Memory management
Key optimization techniques:
- Controlled vs Uncontrolled components
- Form state isolation
- Efficient validation strategies
- Memory leak prevention
// components/optimized-form/use-debounced-validation.ts
import { useCallback, useEffect } from "react";
import { useForm } from "react-hook-form";
import debounce from "lodash/debounce";
export function useDebouncedValidation(
callback: () => Promise<void>,
delay = 500
) {
const debouncedCallback = useCallback(debounce(callback, delay), [
callback,
delay,
]);
useEffect(() => {
return () => {
debouncedCallback.cancel();
};
}, [debouncedCallback]);
return debouncedCallback;
}
// components/optimized-form/async-select.tsx
import { useQuery } from "@tanstack/react-query";
import { useState, useMemo } from "react";
import { Select } from "../ui/select";
interface AsyncSelectProps {
queryKey: string;
queryFn: () => Promise<any[]>;
label: string;
name: string;
}
export function AsyncSelect({
queryKey,
queryFn,
label,
name,
}: AsyncSelectProps) {
const [search, setSearch] = useState("");
const { data, isLoading } = useQuery([queryKey, search], queryFn);
const filteredOptions = useMemo(() => {
if (!data) return [];
return data.filter((option) =>
option.label.toLowerCase().includes(search.toLowerCase())
);
}, [data, search]);
return (
<Select
label={label}
name={name}
options={filteredOptions}
isLoading={isLoading}
onSearch={setSearch}
filterOption={false}
/>
);
}
Accessibility & UX
Accessible Form Components
Accessibility is essential for modern web applications:
- ARIA attributes
- Keyboard navigation
- Screen reader support
- Focus management
// components/ui/form-control.tsx
import * as React from "react";
import { useFormContext } from "react-hook-form";
import { cn } from "@/lib/utils";
interface FormControlProps extends React.HTMLAttributes<HTMLDivElement> {
name: string;
label: string;
helperText?: string;
children: React.ReactNode;
}
export function FormControl({
name,
label,
helperText,
children,
className,
...props
}: FormControlProps) {
const {
formState: { errors },
} = useFormContext();
const error = errors[name];
const id = `form-control-${name}`;
const helperId = `${id}-helper`;
const errorId = `${id}-error`;
return (
<div
role="group"
aria-labelledby={id}
className={cn("space-y-2", className)}
{...props}
>
<label
id={id}
htmlFor={name}
className={cn("block text-sm font-medium", error && "text-destructive")}
>
{label}
</label>
{React.cloneElement(children as React.ReactElement, {
id: name,
"aria-describedby": error ? errorId : helperText ? helperId : undefined,
"aria-invalid": error ? "true" : undefined,
})}
{(helperText || error) && (
<p
id={error ? errorId : helperId}
className={cn(
"text-sm",
error ? "text-destructive" : "text-muted-foreground"
)}
>
{error ? error.message?.toString() : helperText}
</p>
)}
</div>
);
}
Server Integration
Server Actions Integration
// app/actions/booking.ts
"use server";
import { z } from "zod";
import { bookingFormSchema } from "@/lib/validations/booking";
import { prisma } from "@/lib/prisma";
import { sendEmail } from "@/lib/email";
export async function createBooking(data: z.infer<typeof bookingFormSchema>) {
try {
// Validate data
const validated = bookingFormSchema.parse(data);
// Create booking in database
const booking = await prisma.booking.create({
data: {
firstName: validated.personalInfo.firstName,
lastName: validated.personalInfo.lastName,
email: validated.personalInfo.email,
phone: validated.personalInfo.phone,
date: validated.appointment.date,
service: validated.appointment.service,
notes: validated.appointment.notes,
reminders: validated.preferences.reminders,
reminderType: validated.preferences.reminderType,
},
});
// Send confirmation email
if (validated.preferences.reminders) {
await sendEmail({
to: validated.personalInfo.email,
subject: "Booking Confirmation",
template: "booking-confirmation",
data: {
name: `${validated.personalInfo.firstName} ${validated.personalInfo.lastName}`,
date: validated.appointment.date,
service: validated.appointment.service,
},
});
}
return { success: true, booking };
} catch (error) {
if (error instanceof z.ZodError) {
return {
success: false,
error: "Validation failed",
details: error.errors,
};
}
console.error("Booking creation failed:", error);
return {
success: false,
error: "Failed to create booking",
};
}
}
Testing Strategy
Form Testing Setup
// __tests__/booking-form.test.tsx
import { render, screen, waitFor } from "@testing-library/react";
import userEvent from "@testing-library/user-event";
import { BookingForm } from "@/components/booking-form";
describe("BookingForm", () => {
const mockSubmit = jest.fn();
beforeEach(() => {
mockSubmit.mockClear();
});
it("validates required fields", async () => {
render(<BookingForm onSubmit={mockSubmit} />);
// Try to submit without filling required fields
await userEvent.click(screen.getByRole("button", { name: /submit/i }));
// Check for error messages
expect(screen.getByText(/first name is required/i)).toBeInTheDocument();
expect(screen.getByText(/email is required/i)).toBeInTheDocument();
expect(mockSubmit).not.toHaveBeenCalled();
});
it("submits form with valid data", async () => {
render(<BookingForm onSubmit={mockSubmit} />);
// Fill form
await userEvent.type(screen.getByLabelText(/first name/i), "John");
await userEvent.type(screen.getByLabelText(/last name/i), "Doe");
await userEvent.type(screen.getByLabelText(/email/i), "john@example.com");
await userEvent.type(screen.getByLabelText(/phone/i), "+1234567890");
// Submit form
await userEvent.click(screen.getByRole("button", { name: /submit/i }));
// Verify submission
await waitFor(() => {
expect(mockSubmit).toHaveBeenCalledWith({
personalInfo: {
firstName: "John",
lastName: "Doe",
email: "john@example.com",
phone: "+1234567890",
},
// ... other form data
});
});
});
});
Real-World Example: Multi-step Booking Form
Let's build a complete booking form system with all the concepts we've covered.
// components/booking/booking-form.tsx
"use client";
import { useMultiStepForm } from "@/hooks/use-multi-step-form";
import { bookingFormSchema } from "@/lib/validations/booking";
import { createBooking } from "@/app/actions/booking";
import { PersonalInfoStep } from "./steps/personal-info";
import { AppointmentStep } from "./steps/appointment";
import { PreferencesStep } from "./steps/preferences";
import { FormProgress } from "./form-progress";
import { FormNavigation } from "./form-navigation";
export function BookingForm() {
const { form, currentStep, next, back, isFirstStep, isLastStep } =
useMultiStepForm({
schema: bookingFormSchema,
onSubmit: createBooking,
});
const steps = [
{
title: "Personal Information",
component: <PersonalInfoStep form={form} />,
},
{
title: "Appointment Details",
component: <AppointmentStep form={form} />,
},
{
title: "Preferences",
component: <PreferencesStep form={form} />,
},
];
return (
<div className="max-w-2xl mx-auto p-6">
<FormProgress steps={steps} currentStep={currentStep} />
<form onSubmit={form.handleSubmit(createBooking)} className="space-y-8">
{steps[currentStep].component}
<FormNavigation
onBack={back}
onNext={next}
isFirstStep={isFirstStep}
isLastStep={isLastStep}
/>
</form>
</div>
);
}
// components/booking/steps/personal-info.tsx
export function PersonalInfoStep({ form }: { form: UseFormReturn }) {
return (
<div className="space-y-4">
<FormField
name="personalInfo.firstName"
label="First Name"
placeholder="John"
/>
<FormField
name="personalInfo.lastName"
label="Last Name"
placeholder="Doe"
/>
<FormField
name="personalInfo.email"
label="Email"
type="email"
placeholder="john@example.com"
/>
<FormField
name="personalInfo.phone"
label="Phone"
type="tel"
placeholder="+1234567890"
/>
</div>
);
}
// components/booking/steps/appointment.tsx
export function AppointmentStep({ form }: { form: UseFormReturn }) {
return (
<div className="space-y-4">
<FormField
name="appointment.date"
label="Date & Time"
type="datetime-local"
/>
<AsyncSelect
name="appointment.service"
label="Service"
queryKey="services"
queryFn={fetchServices}
/>
<FormField
name="appointment.notes"
label="Additional Notes"
multiline
rows={4}
placeholder="Any special requirements..."
/>
</div>
);
}
// components/booking/steps/preferences.tsx
export function PreferencesStep({ form }: { form: UseFormReturn }) {
const { watch } = form;
const reminders = watch("preferences.reminders");
return (
<div className="space-y-4">
<FormField
name="preferences.reminders"
label="Enable Reminders"
type="checkbox"
/>
{reminders && (
<FormField
name="preferences.reminderType"
label="Reminder Type"
type="radio"
options={[
{ label: "Email", value: "email" },
{ label: "SMS", value: "sms" },
]}
/>
)}
<FormField
name="preferences.marketingConsent"
label="Receive marketing updates"
type="checkbox"
helperText="We'll send you occasional updates about our services"
/>
</div>
);
}
Best Practices & Common Pitfalls
-
Form State Management
- Use controlled components sparingly
- Implement proper form reset
- Handle loading and error states
-
Validation
- Validate on blur for better UX
- Implement server-side validation
- Show clear error messages
-
Performance
- Debounce validation for expensive operations
- Lazy load complex form components
- Optimize re-renders
-
Accessibility
- Proper ARIA attributes
- Keyboard navigation
- Clear error announcements
-
Security
- Sanitize inputs
- Implement CSRF protection
- Rate limiting for submissions
Conclusion
Building robust forms requires attention to detail in validation, accessibility, performance, and user experience. This guide provides a solid foundation for implementing complex forms in Next.js applications.