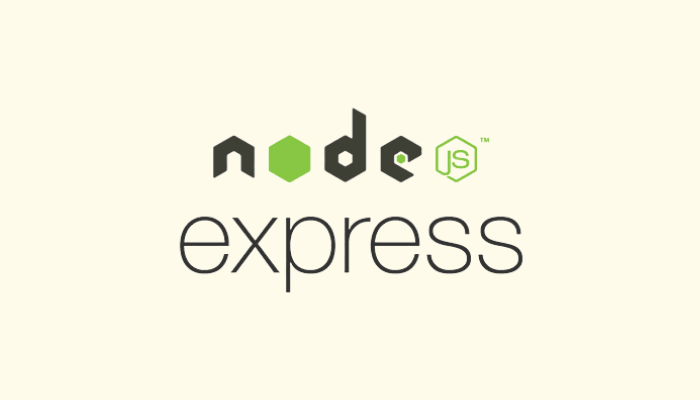
Mastering Express.js: The Complete Guide to Building Professional APIs
Table of Contents
- Introduction
- Diving Deeper: Core Concepts
- Middleware Architecture
- Routing & Controllers
- Error Handling
- Database Integration
- Authentication & Authorization
- API Security
- Performance Optimization
- Testing Strategies
- Real-World Implementation
- Best Practices & Patterns
Introduction
Express.js stands as the cornerstone of Node.js web development, offering a minimalist yet powerful framework for building scalable web applications and APIs. While its simplicity makes it accessible, mastering Express requires understanding its nuanced architecture, middleware system, and best practices for production deployment.
This comprehensive guide will take you from understanding the core principles to implementing advanced patterns, ensuring you can build robust, secure, and maintainable applications. We'll explore why certain patterns emerge, when to use specific features, and how to avoid common pitfalls that plague many Express applications in production.
Why Choose Express.js?
1. Minimalist and Flexible
Express.js is designed to be minimal and unopinionated, allowing developers to structure their applications as they see fit. This flexibility is particularly beneficial for building APIs, as it enables you to choose the components and middleware that best suit your needs.
2. Middleware Support
One of the standout features of Express is its middleware architecture. Middleware functions are functions that have access to the request and response objects, allowing you to modify the request, end the response, or call the next middleware function in the stack. This makes it easy to add functionality such as logging, authentication, and error handling.
3. Robust Routing
Express provides a powerful routing mechanism that allows you to define routes for your API endpoints easily. You can create complex routing structures with parameters, query strings, and more, making it simple to handle various HTTP methods and paths.
4. Large Ecosystem
With a vast ecosystem of third-party middleware and plugins, Express allows you to extend its functionality effortlessly. Whether you need to connect to a database, handle file uploads, or implement authentication, there’s likely a middleware package available to help.
Getting Started with Express
Installation
To get started with Express, you need to have Node.js installed on your machine. Once you have Node.js set up, you can create a new project and install Express using npm:
mkdir express-api
cd express-api
npm init -y
npm install express
Creating Your First Express Application
Let’s create a simple Express application to understand its basic structure. Create a file named app.js
and add the following code:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
// Middleware to parse JSON bodies
app.use(express.json());
// Basic route
app.get('/', (req, res) => {
res.send('Welcome to the Express API!');
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Running the Application
To run your application, use the following command:
node app.js
You should see a message indicating that the server is running. Open your browser and navigate to http://localhost:3000
to see the welcome message.
Core Concepts of Express
Middleware
Middleware functions are the backbone of Express applications. They can perform a variety of tasks, such as logging requests, handling errors, and serving static files. Here’s an example of a simple logging middleware:
app.use((req, res, next) => {
console.log(`${req.method} ${req.url}`);
next(); // Call the next middleware
});
Routing
Routing in Express allows you to define how your application responds to client requests. You can define routes for different HTTP methods (GET, POST, PUT, DELETE) and specify route parameters. Here’s an example of defining routes:
app.get('/users', (req, res) => {
// Logic to retrieve users
res.json([{ id: 1, name: 'John Doe' }]);
});
app.post('/users', (req, res) => {
const newUser = req.body; // Get user data from request body
// Logic to save the user
res.status(201).json(newUser);
});
Error Handling
Error handling is crucial for any API. Express provides a simple way to handle errors using middleware. Here’s an example of an error-handling middleware:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
Serving Static Files
Express can serve static files such as images, CSS, and JavaScript files. You can use the built-in express.static
middleware to serve static files from a directory:
app.use(express.static('public'));
Building a Real-World API with Express
Now that we have covered the basics, let’s build a more comprehensive API for managing a simple task list. This API will allow users to create, read, update, and delete tasks.
Step 1: Setting Up the Project
Create a new directory for your task list API and initialize a new Node.js project:
mkdir task-list-api
cd task-list-api
npm init -y
npm install express
Step 2: Creating the API
Create a file named server.js
and add the following code:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(express.json());
let tasks = []; // In-memory task storage
// GET all tasks
app.get('/tasks', (req, res) => {
res.json(tasks);
});
// POST a new task
app.post('/tasks', (req, res) => {
const newTask = { id: tasks.length + 1, ...req.body };
tasks.push(newTask);
res.status(201).json(newTask);
});
// PUT to update a task
app.put('/tasks/:id', (req, res) => {
const taskId = parseInt(req.params.id);
const taskIndex = tasks.findIndex(task => task.id === taskId);
if (taskIndex === -1) {
return res.status(404).send('Task not found');
}
tasks[taskIndex] = { id: taskId, ...req.body };
res.json(tasks[taskIndex]);
});
// DELETE a task
app.delete('/tasks/:id', (req, res) => {
const taskId = parseInt(req.params.id);
tasks = tasks.filter(task => task.id !== taskId);
res.status(204).send();
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Step 3: Testing the API
You can test your API using tools like Postman or cURL. Here are some example requests:
- Get all tasks:
GET http://localhost:3000/tasks
- Create a new task:
POST http://localhost:3000/tasks
with JSON body{" title": "Learn Express", "completed": false}
- Update a task:
PUT http://localhost:3000/tasks/1
with JSON body{" title": "Learn Express.js", "completed": true}
- Delete a task:
DELETE http://localhost:3000/tasks/1
Diving Deeper: Core Concepts
Application Setup
Let's start with a properly structured Express application:
// src/app.ts
import express, { Application } from "express";
import compression from "compression";
import cors from "cors";
import helmet from "helmet";
import morgan from "morgan";
import { errorHandler } from "./middleware/error.middleware";
import { notFoundHandler } from "./middleware/notFound.middleware";
import { routes } from "./routes";
import { config } from "./config";
export class App {
public express: Application;
constructor() {
this.express = express();
this.initializeMiddleware();
this.initializeRoutes();
this.initializeErrorHandling();
}
private initializeMiddleware(): void {
// Security middleware
this.express.use(helmet());
this.express.use(cors(config.cors));
// Request parsing
this.express.use(express.json());
this.express.use(express.urlencoded({ extended: true }));
// Performance middleware
this.express.use(compression());
// Logging
if (config.env !== "test") {
this.express.use(morgan("combined"));
}
// Request ID tracking
this.express.use((req, res, next) => {
req.id = crypto.randomUUID();
next();
});
}
private initializeRoutes(): void {
this.express.use("/api", routes);
}
private initializeErrorHandling(): void {
this.express.use(notFoundHandler);
this.express.use(errorHandler);
}
}
export default new App().express;
Configuration Management
Proper configuration management is crucial for maintainable applications:
// src/config/index.ts
import dotenv from "dotenv";
import { z } from "zod";
// Load environment variables
dotenv.config();
// Configuration schema
const configSchema = z.object({
env: z.enum(["development", "production", "test"]),
port: z.number().min(1).max(65535),
database: z.object({
url: z.string().url(),
maxConnections: z.number().positive(),
ssl: z.boolean(),
}),
jwt: z.object({
secret: z.string().min(32),
expiresIn: z.string(),
}),
cors: z.object({
origin: z.union([z.string(), z.array(z.string())]),
credentials: z.boolean(),
}),
rateLimit: z.object({
windowMs: z.number(),
max: z.number(),
}),
});
// Parse and validate configuration
export const config = configSchema.parse({
env: process.env.NODE_ENV || "development",
port: parseInt(process.env.PORT || "3000", 10),
database: {
url: process.env.DATABASE_URL,
maxConnections: parseInt(process.env.DB_MAX_CONNECTIONS || "10", 10),
ssl: process.env.DB_SSL === "true",
},
jwt: {
secret: process.env.JWT_SECRET,
expiresIn: process.env.JWT_EXPIRES_IN || "1d",
},
cors: {
origin: process.env.CORS_ORIGIN?.split(",") || "*",
credentials: true,
},
rateLimit: {
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
},
});
Middleware Architecture
Understanding middleware is crucial as it's the backbone of Express applications. Here's a comprehensive look at different middleware types and their implementations:
Authentication Middleware
// src/middleware/auth.middleware.ts
import { Request, Response, NextFunction } from "express";
import jwt from "jsonwebtoken";
import { config } from "../config";
import { UserService } from "../services/user.service";
import { ApiError } from "../utils/ApiError";
export interface AuthRequest extends Request {
user?: any;
}
export class AuthMiddleware {
static authenticate = async (
req: AuthRequest,
res: Response,
next: NextFunction
) => {
try {
const token = AuthMiddleware.extractToken(req);
if (!token) {
throw new ApiError(401, "No token provided");
}
const decoded = jwt.verify(token, config.jwt.secret);
const user = await UserService.findById(decoded.sub);
if (!user) {
throw new ApiError(401, "User not found");
}
req.user = user;
next();
} catch (error) {
if (error instanceof jwt.JsonWebTokenError) {
next(new ApiError(401, "Invalid token"));
} else {
next(error);
}
}
};
static authorize = (...roles: string[]) => {
return (req: AuthRequest, res: Response, next: NextFunction) => {
if (!req.user) {
throw new ApiError(401, "Not authenticated");
}
if (!roles.includes(req.user.role)) {
throw new ApiError(403, "Insufficient permissions");
}
next();
};
};
private static extractToken(req: Request): string | null {
if (
req.headers.authorization &&
req.headers.authorization.startsWith("Bearer ")
) {
return req.headers.authorization.split(" ")[1];
}
if (req.cookies && req.cookies.token) {
return req.cookies.token;
}
return null;
}
}
Request Validation
// src/middleware/validation.middleware.ts
import { Request, Response, NextFunction } from "express";
import { AnyZodObject, ZodError } from "zod";
import { ApiError } from "../utils/ApiError";
export const validate =
(schema: AnyZodObject) =>
async (req: Request, res: Response, next: NextFunction) => {
try {
await schema.parseAsync({
body: req.body,
query: req.query,
params: req.params,
});
next();
} catch (error) {
if (error instanceof ZodError) {
next(
new ApiError(400, "Validation Error", {
errors: error.errors.map((e) => ({
path: e.path.join("."),
message: e.message,
})),
})
);
} else {
next(error);
}
}
};
// Usage example
const createUserSchema = z.object({
body: z.object({
email: z.string().email(),
password: z.string().min(8),
name: z.string().min(2),
}),
});
router.post("/users", validate(createUserSchema), userController.create);
Error Handling
// src/middleware/error.middleware.ts
import { Request, Response, NextFunction } from "express";
import { ApiError } from "../utils/ApiError";
import { logger } from "../utils/logger";
export const errorHandler = (
error: Error,
req: Request,
res: Response,
next: NextFunction
) => {
logger.error({
message: error.message,
stack: error.stack,
requestId: req.id,
path: req.path,
method: req.method,
});
if (error instanceof ApiError) {
return res.status(error.statusCode).json({
status: "error",
message: error.message,
errors: error.errors,
code: error.statusCode,
});
}
// Handle specific error types
if (error.name === "ValidationError") {
return res.status(400).json({
status: "error",
message: "Validation Error",
errors: error.errors,
code: 400,
});
}
if (error.name === "UnauthorizedError") {
return res.status(401).json({
status: "error",
message: "Unauthorized",
code: 401,
});
}
// Default error
return res.status(500).json({
status: "error",
message: "Internal Server Error",
code: 500,
});
};
Request Logging
// src/middleware/logging.middleware.ts
import { Request, Response, NextFunction } from "express";
import { logger } from "../utils/logger";
export const requestLogger = (
req: Request,
res: Response,
next: NextFunction
) => {
const startTime = process.hrtime();
// Log request
logger.info({
type: "request",
id: req.id,
method: req.method,
path: req.path,
query: req.query,
headers: {
"user-agent": req.get("user-agent"),
"content-type": req.get("content-type"),
},
});
// Log response
res.on("finish", () => {
const [seconds, nanoseconds] = process.hrtime(startTime);
const duration = seconds * 1000 + nanoseconds / 1000000;
logger.info({
type: "response",
id: req.id,
status: res.statusCode,
duration: `${duration.toFixed(2)}ms`,
});
});
next();
};
Routing & Controllers
Implementing a clean and maintainable routing structure:
Route Setup
// src/routes/index.ts
import { Router } from "express";
import userRoutes from "./user.routes";
import authRoutes from "./auth.routes";
import productRoutes from "./product.routes";
const router = Router();
router.use("/auth", authRoutes);
router.use("/users", userRoutes);
router.use("/products", productRoutes);
export { router };
// src/routes/user.routes.ts
import { Router } from "express";
import { UserController } from "../controllers/user.controller";
import { AuthMiddleware } from "../middleware/auth.middleware";
import { validate } from "../middleware/validation.middleware";
import { userSchemas } from "../schemas/user.schema";
const router = Router();
const controller = new UserController();
router
.route("/")
.get(
AuthMiddleware.authenticate,
AuthMiddleware.authorize("admin"),
controller.getAll
)
.post(validate(userSchemas.createUser), controller.create);
router
.route("/:id")
.get(
AuthMiddleware.authenticate,
validate(userSchemas.getUser),
controller.getById
)
.put(
AuthMiddleware.authenticate,
validate(userSchemas.updateUser),
controller.update
)
.delete(
AuthMiddleware.authenticate,
AuthMiddleware.authorize("admin"),
validate(userSchemas.deleteUser),
controller.delete
);
export default router;
Controller Implementation
// src/controllers/base.controller.ts
import { Request, Response, NextFunction } from "express";
import { ApiError } from "../utils/ApiError";
export abstract class BaseController {
protected async executeImpl(
req: Request,
res: Response,
next: NextFunction
): Promise<void | any> {
try {
await this.execute(req, res, next);
} catch (error) {
next(error);
}
}
protected abstract execute(
req: Request,
res: Response,
next: NextFunction
): Promise<void | any>;
protected ok<T>(res: Response, dto?: T) {
if (dto) {
return res.status(200).json({
status: "success",
data: dto,
});
}
return res.sendStatus(200);
}
protected created<T>(res: Response, dto?: T) {
if (dto) {
return res.status(201).json({
status: "success",
data: dto,
});
}
return res.sendStatus(201);
}
protected clientError(message?: string) {
return new ApiError(400, message || "Bad request");
}
protected unauthorized(message?: string) {
return new ApiError(401, message || "Unauthorized");
}
protected forbidden(message?: string) {
return new ApiError(403, message || "Forbidden");
}
protected notFound(message?: string) {
return new ApiError(404, message || "Not found");
}
}
// src/controllers/user.controller.ts
import { Request, Response, NextFunction } from "express";
import { BaseController } from "./base.controller";
import { UserService } from "../services/user.service";
import { PaginationParams } from "../types/pagination";
export class UserController extends BaseController {
constructor(private userService = new UserService()) {
super();
}
getAll = async (req: Request, res: Response, next: NextFunction) => {
const pagination: PaginationParams = {
page: parseInt(req.query.page as string) || 1,
limit: parseInt(req.query.limit as string) || 10,
};
const filters = {
role: req.query.role as string,
status: req.query.status as string,
};
const users = await this.userService.findAll(pagination, filters);
this.ok(res, users);
};
getById = async (req: Request, res: Response, next: NextFunction) => {
const user = await this.userService.findById(req.params.id);
if (!user) {
throw this.notFound("User not found");
}
this.ok(res, user);
};
create = async (req: Request, res: Response, next: NextFunction) => {
const user = await this.userService.create(req.body);
this.created(res, user);
};
update = async (req: Request, res: Response, next: NextFunction) => {
const user = await this.userService.update(req.params.id, req.body);
if (!user) {
throw this.notFound("User not found");
}
this.ok(res, user);
};
delete = async (req: Request, res: Response, next: NextFunction) => {
await this.userService.delete(req.params.id);
this.ok(res);
};
}
Best Practices & Patterns
-
Error Handling
- Use custom error classes
- Implement global error handling
- Provide meaningful error messages
- Log errors appropriately
-
Security
- Implement rate limiting
- Use security headers
- Validate input data
- Implement proper authentication
- Use HTTPS
- Implement CORS properly
-
Performance
- Use caching strategies
- Implement database indexing
- Use compression
- Optimize database queries
- Implement connection pooling
-
Code Organization
- Follow SOLID principles
- Use dependency injection
- Implement proper layering
- Use TypeScript for type safety
-
Testing
- Write unit tests
- Implement integration tests
- Use test coverage tools
- Mock external services
Conclusion
Building robust Express.js applications requires understanding various concepts and implementing them correctly. This guide covered essential aspects from basic setup to advanced patterns and security considerations. By following these practices and patterns, you can build scalable, maintainable, and secure Express.js applications.
Remember to:
- Always validate input
- Handle errors gracefully
- Implement proper security measures
- Monitor performance
- Write tests
- Document your code
- Keep dependencies updated